Robocode Robot S

In this lesson, we describe the basics of how the scanning works.
Aug 27, 2010 This means that Robocode will help adjusting the gun when fire is called so it will be better at hitting enemies seen when scanning a robot. The fire assistance is only available for Robots, and is a 'compensation' for all the disadvantages a Robot has compared to an AdvancedRobot. The Robocode Tutorials Information Introduction to Robocode Guide to Robocode. Tutorials My Second Robot Figure8 SuperCorners SuperWalls Bullet-dodging SharpShooter. Appendices Index of Topics by Sample Robot Sample Robots source.
Your Lab 3 exercise correpsonds to thisrobocode lesson.
Robot Senses
We'll begin this lesson by discussing your robot's senses. It has only afew.
Sense of Touch
Your robot knows when it's:
- hit a wall (onHitWall),
- been hit by a bullet (onHitByBullet),
- or hit another robot (onHitRobot).
All of these methods pass you events that give you information about whatyou touched.
Sense of Sight
Your robot knows when it's seen another robot, but only if it scans it (onScannedRobot).
Scan events are arguably the most important of all events. Scan eventsgive you information about the other robots on the battlefield. (Some robotsput 90+% of their code in the onScannedRobot method.) The only wayscan events can be generated (practically speaking) is if you move your radar.(If an enemy robot wanders in front of your radar it will generate a scanevent, but you should really take a more proactive stance.)
Also, remember per Lesson 2, the scanner isthe fastest moving part of your robot, so don't be stingy about moving it.
If you want to, you can make the robots' scan arcs visible by selectingOptions Menu -> Preferences -> View Options Tab and click on the'Visible Scan Arcs' checkbox. This is handy when debugging.
Other Senses
Your robot also knows when he's died (onDeath), when another robot has died (onRobotDeath -- we will use this one today), or when he's won the round (onWin -- this is where you write the code for your victory dance).
Your robot also is aware of his bullets and knows when a bullet has hit an opponent (onBulletHit), when a bullet hits a wall (onBulletMissed), when a bullet hits another bullet (onBulletHitBullet).
Building A Better Robot
Here's some basic scanning movement. Note again that we call setAdjustRadarForRobotTurn(true) so as to have independent radar movement.
Lagu OST Master's Sun Lirik Lagu Master Sun OST RCTI Touch Love - Yoon Mi-rae Touch Love adalah lagu OST Master's Sun yang dibawakan oleh penyanyi bernama Yoon Mirae. Atau kita juga mengenalnya dengan nama Tasya Reid yang tergabung dalam Grup MFBTY yang merupakan singkatan 'My Fans Better Than Yours' Grup Musik dari Korea. Drama “Descendants of the Sun” tidak hanya memiliki alur cerita yang menarik dan pemain-pemain yang populer, pemirsa setia drama ini juga dibuat jatuh hati dengan original soundtrack (OST) yang spektakuler. Mulai dari lagu ballad hingga upbeat, lagu-lagu dalam OST ini mampu memikat dan menyentuh perasaanmu. Lagu-lagu ini juga mendominasi hampir semua tangga lagu di Korea, bersaing dengan. Download lagu sontrek drama korea master sun. Free Download Korean Series Drama (118.44 MB) Download Lagu Korea Master Sun Mp3 Gratis, Free Download Korea Master Sun Mp3, Unduh Lagu Korea Master Sun Mp3 Secara Percuma. Horrrrreeeee preview Episode terakhir sepertinya bakal happyy ending?.Walaupun TGS kayaknya hilang ingatan? Baiklah sekarang mau share OSTnya?
Serial Movements with The Robot Class
You want to find other robots to kill. To do that, you need to scan forother robots. The simplest approach to scanning is to just turn the radararound and around. Your run method could look something like this:
Indeed, our beloved BearingBotfrom last week did exactly that: he rotates his radar and moves closer towhoever he scans.
You may have noticed that BearingBot has a large defect that you can seeif you match him up against an opponent that moves a lot (like, say, Crazy).He scans an opponent, then moves to where he saw him, and by the time he getsthere, the opponent has moved away.
Compound Movements with the AdvancedRobot Class
It would be great if we could do more than one thing at once (scan ANDturn AND fire). Thankfully, the powers that be have provided us with a meansto accomplish this: The AdvancedRobotbase class, which allows us to make non-blocking calls to move the robot andthen executes them all as a compound action. Crazy and SpinBot (and oddlyenough SittingDuck) are all examples of advanced robots.
To change your robot to an advanced robot, just change your classdeclaration from:toNow you're inheriting from AdvancedRobot rather than Robot;now you can use the set* methods provided by the AdvancedRobot class.(We will discuss more about inheritance when we cover chapter 5.)
Sample robot:AdvancedBearingBot a great improvement over 'BearingBot', because hecan do compound movements.
Sample robot:AdvancedTracker - This is a modification of the 'Tracker' sample robot.Per the source code for Tracker, notice how much he improves when you turn himinto an AdvancedRobot.
Important Note: If you make a robot derived from AdvancedRobot, youmust call the execute() method to perform all the queued-up actions.
But AdvancedBearingBot has another large defect which you can see if youmatch him up against more than one opponent: he goes driving all over thebattlefield chasing one robot after another and doesn't get a lotaccomplished. This is because his radar keeps scanning robots, and he chasesevery one he scans. In short, he lacks focus.
Locking Onto an Enemy
Narrow Beam
We can easily lock onto our opponent by constantly turning the radartoward him whenever we scan him. Intuatively, you might think of doingsomething with the scanned robot's bearing like so:
There's a problem with this, though: the ScannedRobotEvent gives us abearing to the scanned robot but it is relative to our tank's position,not our radar's position. How do we resolve this little quandry?
Easy: we find the difference between our tank heading (getHeading())and our radar heading (getRadarHeading())and add the bearing to the scanned robot (e.getBearing()),like so:
Sample robot:NarrowBeam - Uses the above source to lock onto an opponent and nail him.Match him up against as many opponents as you want.
A recurring theme in the computer industry is that the solution to oneproblem leads to the creation of another. Fittingly, having solved the problemof how to lock onto a target, we are now faced with another problem, which thefollowing screenshot illustrates:
Note that NarrowBeam has locked on so tightly to Crazy that he is blithelyunaware that Tracker is killing him from behind.
Oscillating (or 'Wobbling') the Radar
In this technique, every time you see an opponent, you whipsaw the radarback so as to focus on one robot and continuously generate scan events. Thisis an improvement over the narrow beam because you are more aware of nearbyrobots.
To make this work, you need a variable that keeps track of which directionto turn the radar, it will only ever have values of 1 and -1, so it can besmall. You can declare it in your robot like so:The run method can look just like the one above, but in theonScannedRobot method you do the following:Flipping the value of scanDirection creates the oscillating effect.
Sample robot: Oscillator -wobbles his radar to keep track of hist opponent. Note that while he tends totrack an enemy, he'll chase others that are nearby, too.
But we still haven't completely solved the problem with NarrowBeam: otherrobots can still sneak up behind Oscillator and shoot him. Also, Oscillatortends to get a little unfocused, at times.
Enemy Tracking
Using the EnemyBot Class
A further improvement that could be made would be to single out anindividual robot, focus on him, and destroy him completely. The sample robotTracker does this to a limited extent, but we'll do one better with the EnemyBot class you guys all wrote.
To keep track of the information about an enemy robot, you first need tomake a member variable in your robot like so:You will want to reset (clear) your enemy at the top of your runmethod like so:And you need to update the enemy's information in the onScannedRobot methodlike so:From this point, you can use all of the information about the enemy in anyother method of your class.
There is one last detail, though, if the enemy you're tracking dies,you'll want to reset it so you can track another. To do that, implement anonRobotDeath method like so:
Sample robot:EnemyTracker - a robot that uses the EnemyBot class. Note that even thoughhe rotates the radar, he just tracks one enemy. This allows him to keep an eyeon what's going on in the rest of the battlefield while concentrating on histarget.
Slightly Smarter Tracking
Another small optimization could be made here: If a closer robot movesinto view, we probably want to start shooting him instead. You can accomplishthis by modifying your onScannedRobot method like so:
Sample robot:EnemyOrCloser uses the above scanning technique to hit the closest enemy.As he rotates his radar, he will begin tracking any enemy that is closer (evenif someone sneaks up behind him).
In this lesson, we describe the basics of turning and aiming your gun.Some of this stuff gets a little heavy, but I think you guys can handle it.
Your Lab 4 exercise correpsonds to thisrobocode lesson.
Ready, Aim, Fire!
Independent Gun Movement
We really made some headway last week with robots like Oscillator and EnemyTracker, but they had a big shortcoming:they all had to be driving toward their enemy to shoot at it. As we recallfrom Lesson #2, your robot consists ofthree parts, all of which can move independently, and the tank part movesslowest of all.
One improvement we could make is to divorce gun movement from robotmovement. This can be done easily by callingsetAdjustGunForRobotTurn(true). Thereafter, you can make calls like turnGunRight() (or better yet setTurnGunRight()) to turn the gun independently. Now you can turn the gunone direction and move the tank a different direction.
Simple Aiming Formula
We can easily turn the gun toward our opponent when we scan him by using aformula similar to the narrow beamscan from last week: we find the difference between our tank heading (getHeading())and our gun heading (getGunHeading())and add the bearing to the target (getBearing()),like so:
Firepower Calculation Formula
Another important aspect of firing is calculating the firepower of yourbullet. The documentation for the fire() method explains that you can fire a bullet in the range of 0.1 to3.0. As you've probably already concluded, it's a good idea to firelow-strength bullets when your enemy is far away, and high-strength bulletswhen he's close.
You could use a series of if-else-if-else statements to determinefirepower, based on whether the enemy is 100 pixels away, 200 pixels away,etc. But such constructs are a bit too rigid. After all, the range ofpossible firepower values falls along a continuum, not discrete blocks.A better approach is to use a formula. Here's an example:With this formula, as the enemy distance increases, the firepower decreases.Likewise, as the enemy gets closer, the firepower gets larger. Values higherthan 3 are floored to 3 so we will never fire a bullet larger than 3, but weshould probably floor the value anyway (just to be on the safe side) like so:
Sample robot:Shooter is arobot that features independent gun movement and uses both of the aboveformulas to shoot at an enemy. Match him up against SittingDuck, Target, Fire,TrackFire, Corners, and maybe even Tracker and watch him spin and shoot.
More Efficient Aiming
As you may have noticed, Shooter has a problem: sometimes he turns his gunbarrel the long way around to aim at an enemy. (Sometimes he just sits therespinning his gun barrel.) Worst case, he might turn his gun 359 degrees to hitan enemy that is 1 degree away from his gun.
Normalized Bearings
The problem is the result of getting a non-normalized bearing fromthe simple aiming formula above. A normalized bearing (like the kindyou get in a ScannedRobotEvent) is a bearing between -180 and +180 degrees asdepicted in the following illustration:
A non-normalized bearing could be smaller than -180 or larger than 180.We like to work with normalized bearings because they make for more efficientmovement. To normalize a bearing, use the following function:Note the use of while statements rather than if statementsto handle cases where the angle passed is extremely large or extremely small.(A while is just like an if, except it loops.)
Sample robot:NormalizedShooter which normalizes the gun turns by using the abovefunction.
Avoiding Premature Shooting
A problem with the Shooter and NormalizedShooter robots above is that theymight fire before they've turned the gun toward the target. Even after younormalize the bearing, you could still fire prematurely.
To avoid premature shooting, call the getGunTurnRemaining() method to see how far away your gun is from thetarget and don't fire until you're close.
Additionally, you cannot fire if the gun is 'hot' from the last shot andcalling fire() (or setFire()) will just waste a turn. Wecan test if the gun is cool by calling getGunHeat().
The following code snippet tests for both of these:(Feel free to test with values other than 10.)
Sample robot:EfficientShooter who uses the normalizeBearing function for moreefficient gun turning and avoids premature shooting by using the above ifstatement.
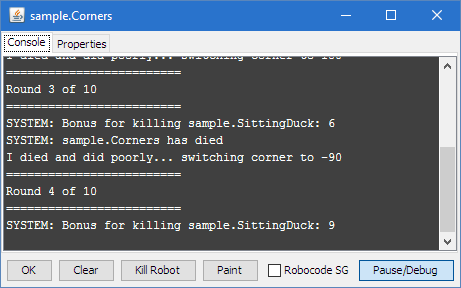
Digression: Absolute Bearings
In contrast to a relative bearing, an absolute bearing is a valuebetween 0 and +360 degrees. The following illustration shows bothe therelative and absolute bearing from one robot to another:
Absolute bearings are often useful. You guys computed an absolute bearingfrom a relative bearing in your AdvancedEnemyBot class to get the x,y coordinates of an enemy.
Another application of absolute bearings is to get the angle between twoarbitrary points. The following function will do this for you:
Sample robot: RunToCentera robot that moves to the center of the battlefield no matter where he startsby getting an absolute bearing between his point and the center of thebattlefield. Note that he normalizes the absolute bearing (by callingnormalizeBearing) for more efficient turning. Match him upagainst Walls to see how one takes the edges, and the other takes thecenter.
Predictive Targting
Or: 'Using Trigonometry to impress your friends and destroy yourenemies
One last problem remains: If you look at how any of the previous robotsfares against Walls, they always miss. The reason this problem occurs isbecause it takes time for the bullet to travel. By the time the bullet getsthere, Walls has already moved on.
If we wanted to be able to hit Walls (or any other robot) more often, we'dneed to be able to predict where he will be in the future, but how can we dothat?
Distance = Rate x Time
Using D = RxT we can figure out how long it will take a bullet to getthere.
- Distance: can be found by calling enemy.getDistance()
- Rate: per the Robocode FAQ, a bullet travels at a rate of 20 - firepower * 3.
- Time: we can compute the time by solving for it:
D = RxT --> T = D/R
Getting Future X,Y Coordinates
Next, we can use the AdvancedEnemyBotclass you guys all wrote, which contains the methods getFutureX() andgetFutureY(). To make use of the new features, we need to change ourcode from:to:Then in the onScannedRobot() method, we need to change the code from:to:
The alert student will note that it is entirely possible to use the oldupdate method, with unfortunate results. Fair warning.
Turning the Gun to the Predicted Point
Lastly, we get the absolute bearing between our tank and the predictedlocation using the absoluteBearing function above. We then find thedifference between the absolute bearing and the current gun heading and turnthe gun, normalizing the turn to take the shortest path there.
Sample robot:PredictiveShooter uses the stuff described above to anticipate where hisenemy will be. Match him up against Walls and watch the magic happen.